Java keywords
Java keywords are reserved and cannot be used as variable names or identifiers, some of commonly used keywords below
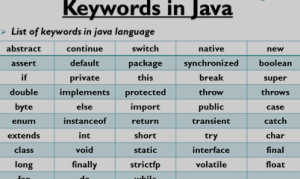
abstract Java Keywords :
Used to declare an abstract class or methods.
Example :
abstract class AbstractClass {
abstract void abstractMethod();
}
boolean:
A data type that can hold True and False values only.
Example : boolean isTrue = true;
break:
Used to break the loop or switch statement.
Example :
for (int i = 0; i < 5; i++) { if (i == 3) { break; }
System.out.println(i);
}
byte:
A data type that can hold 8-bit data values.
Example : byte b = 10
case:
Used in switch statements to mark blocks of text.
Example :
int num = 2;
switch (num) {
case 1:
System.out.println(“One”);
break;
case 2:
System.out.println(“Two”);
break;
}
catch:
Used to catch the exceptions generated by try statements.
Example :
try {
int[] arr = new int[2];
System.out.println(arr[3]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Array index out of bounds”);
}
char:
A data type that can hold unsigned 6-bit Unicode characters.
Example : char c = ‘A’;
class:
Used to declare a class.
Example :
class MyClass {}
continue:
Used to continue the loop.
Example :
for (int i = 0; i < 5; i++) {
if (i == 3) {
continue;
}
System.out.println(i);
}
default:
Specifies the default block of code in a switch statement.
Example :
char grade = ‘B’;
switch (grade) {
case ‘A’:
System.out.println(“Excellent”);
break;
default:
System.out.println(“Good”);
break;
}
do:
Starts a do-while loop.
Example :
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
// 12. double
double d = 10.5;
double:
A data type that can hold 64-bit floating-point numbers.
Example : double d = 10.5;
else:
Indicates alternative branches in an if statement.
Example :
if (isTrue) {
System.out.println(“It’s true”);
} else {
System.out.println(“It’s false”);
}
enum:
Used to declare an enumerated type.
Example : enum Days {SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY}
extends:
Indicates that a class is derived from another class or interface.
Example :
class Parent {}
class Child extends Parent {}
final:
Indicates that a variable holds a constant value or that a method will not be overridden.
Example :
final double PI = 3.14159;
// 17. finally (used with try-catch to execute important code)
try {
int x = 10 / 0;
} catch (ArithmeticException e) {
System.out.println(“Division by zero”);
} finally {
System.out.println(“This will always be printed”);
}
finally:
Indicates a block of code in a try-catch structure that will always be executed.
Example :
float:
A data type that holds a 32-bit floating-point number.
Example : float f = 10.5f;
for:
Used to start a for loop.
Example :
for (int j = 0; j < 5; j++) {
System.out.println(j);
}
if:
Tests a true/false expression and branches accordingly.
Example :
if (isTrue) {
System.out.println(“It’s true”);
}
Java Keywords implements:
Specifies that a class implements an interface.
Example :
interface MyInterface {}
class MyClass implements MyInterface {}
import:
References other classes.
Example : import java.util.ArrayList;
instanceof:
Indicates whether an object is an instance of a specific class or implements an interface.
Example :
String s = “Hello”;
boolean check = s instanceof String;
System.out.println(check); // Outputs true
int:
A data type that can hold a 32-bit signed integer.
Example : int num = 10;
interface:
Declares an interface.
Example :
interface MyInterface {
void myMethod();
}
long:
A data type that holds a 64-bit integer.
Example : long l = 100000L;
native:
Specifies that a method is implemented with native (platform-specific) code.
Example :
new:
Creates new objects.
Example : MyClass obj = new MyClass();
Java Keywords null:
This indicates that a reference does not refer to anything.
Example : MyClass obj2 = null;
package:
Declares a Java package.
Example :
private:
An access specifier indicating that a method or variable may be accessed only in the class it’s declared in.
Example : private int myVar;