Java Abstract Classes
An Java abstract class in Java is a class that cannot be instantiated, meaning you cannot create an object of an abstract class. It is used to declare common characteristics of subclasses. An abstract class can have abstract methods (methods without bodies) as well as methods with implementation.
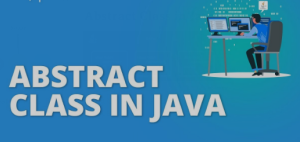
Example:
abstract class Vehicle {
abstract void run();
}
What is an Abstract Class?
In Java, an abstract class is a class that is declared with the keyword `abstract`. It may or may not include abstract methods (methods declared without an implementation). Abstract classes cannot be instantiated, but they can be subclassed.
Why use Abstract Classes?
Java Abstract classes are primarily used for inheritance, where other classes can extend the abstract class, inheriting its properties and methods. The abstract class acts as a blueprint for other classes.
Abstract Methods
An abstract method is a method that is declared without an implementation. It contains just the method signature. The implementation is provided by the subclass.
Example :
abstract class Vehicle {
abstract void run();
}
Concrete Classes
A concrete class in Java is a subclass of an Java abstract class which provides the implementations for all of the abstract methods in its superclass.
Example :
class Car extends Vehicle {
void run() {
System.out.println(“Car is running”);
}
}
Can we instantiate an Abstract Class?
No, we cannot instantiate an Java abstract class. This means you cannot create an object of the abstract class because it’s incomplete. Its implementation is provided by the subclass.
Example :
Vehicle v = new Vehicle(); // Invalid
Vehicle v = new Car(); // Valid
Can an Abstract Class have a Constructor?
Yes, an abstract class can have a constructor in Java. It looks like a normal class constructor but it’s used to initialize the abstract class fields.
Example :
abstract class Vehicle {
Vehicle() {
System.out.println(“Vehicle Constructor”);
}
abstract void run();
}
class Car extends Vehicle {
Car() {
super();
System.out.println(“Car Constructor”);
}
void run() {
System.out.println(“Car is running”);
}
}
In the above example, even though Vehicle is an abstract class, it has a constructor. It gets called when we create an instance of the subclass.
Can an Abstract Class have a Main Method?
Yes, an abstract class can have the main method. It’s static so we don’t need to create an object to call the main method.
Example :
abstract class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
Conclusion
Abstract classes are a powerful feature in Java. They allow you to define a general behavior in a superclass and let subclasses provide the specific implementation. This makes your code more organized and easier to manage. So, unleash the power of abstract classes in your Java programming journey!