Java Object-Oriented Programming
Object-oriented programming (OOP) is a computer programming model that organizes software design around data, or objects, rather than functions and logic. An object can be defined as a data field that has unique attributes and behavior.
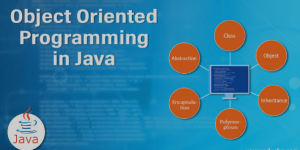
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that uses “objects” – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs.
Four Pillars of Java Object-Oriented Programming
OOP in Java is built around four main principles: Encapsulation, Inheritance, Polymorphism, and Abstraction.
Encapsulation
Encapsulation is the technique of making the fields in a class private and providing access to the fields via public methods. It enhances security as it hides the data.
Example:
public class Employee {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Inheritance
Inheritance is a mechanism where you can derive a class from another class for a hierarchy of classes that share a set of attributes and methods.
Example:
class Vehicle {
void run() {
System.out.println(“Vehicle is running”);
}
}
class Bike extends Vehicle {
void run() {
System.out.println(“Bike is running safely”);
}
}
Polymorphism
Polymorphism allows us to perform a single action in different ways. In Java, we use method overloading and method overriding to achieve polymorphism.
Example:
class Animal {
void sound() {
System.out.println(“The animal makes a sound”);
}
}
class Cat extends Animal {
void sound() {
System.out.println(“The cat meows”);
}
}
Abstraction
Abstraction is a process of hiding the implementation details and showing only functionality to the user.
Example:
abstract class Shape {
abstract void draw();
}
class Rectangle extends Shape {
void draw() {
System.out.println(“Drawing rectangle”);
}
}
some more advanced concepts in Java Object-Oriented Programming (OOP):
Association:
It represents a relationship between two or more objects where all objects have their own lifecycle and there is no owner. The name of an association specifies the nature of the relationship between objects.
Aggregation:
It is a specialized form of Association where all objects have their own lifecycle, but there is ownership, and child objects cannot belong to another parent object.
Composition:
It is a specialized form of Aggregation. It is a strong type of Aggregation. Child objects
do not have their lifecycle, so when the parent object is deleted, all child objects will also be deleted.
Coupling:
Coupling refers to the knowledge or information or dependency of another class. It arises when classes are aware of each other.
Cohesion:
Cohesion refers to what the class (or module) can do. Low cohesion would mean that the class does a great variety of actions – it is broad, unfocused on what it should do. High cohesion means that the class is focused on what it should be doing, i.e., only methods relating to the intention of the class.
IS-A Relationship:
In Java, a class IS-A relationship is based on inheritance or interface. The IS-A relationship is a way of saying, “this object is a type of that object.
HAS-A Relationship:
In Java, a class HAS-A relationship is based on usage, rather than inheritance. In other words, class A HAS-A relationship with class B if code in class A has a reference to an instance of class B.
Method Overloading: If a class has multiple methods having the same name but different in parameters, it is known as Method Overloading.
Method Overriding: If a subclass provides a specific implementation of a method that is already provided by its parent class, it is known as Method Overriding.
Object Cloning: In Java, a class can implement the Cloneable interface to indicate to the Object.clone() method that it is legal for that method to make a field-for-field copy of instances of that class.
Conclusion
Object-Oriented Programming makes development and maintenance easier, as well as providing a number of other benefits. Understanding these concepts are key to becoming a successful Java programmer.