Differences Between Interfaces and Abstract Classes
When it comes to designing object-oriented systems in programming, two key concepts play a vital role in defining the structure of classes and their relationships: interfaces and abstract classes. While they share similarities, they serve different purposes and have distinct characteristics. In this Article, we’ll explore the core concepts of interfaces and abstract classes, highlighting their differences through detailed examples.
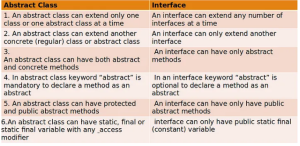
Abstract Classes:
Core Concepts:
Abstraction:
- Abstract classes provide a way to create incomplete classes that cannot be instantiated on their own. They are meant to be extended by concrete (non-abstract) classes.
Methods:
- Abstract classes can contain both abstract methods (methods without a body) and concrete methods (methods with a body). Concrete methods may provide a default implementation that can be inherited by subclasses.
Constructors:
- Abstract classes can have constructors, which are invoked when a subclass is instantiated. This allows for common initialization logic to be shared among subclasses.
Example:
abstract class Shape {
// Abstract method
public abstract double calculateArea();
// Concrete method
public void display() {
System.out.println(“This is a shape.”);
}
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
// Implementation of abstract method
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
class Square extends Shape {
private double side;
public Square(double side) {
this.side = side;
}
// Implementation of abstract method
@Override
public double calculateArea() {
return side * side;
}
}
Interfaces:
Core Concepts:
Multiple Inheritance:
- Unlike abstract classes, interfaces support multiple inheritance. A class can implement multiple interfaces, allowing for greater flexibility in structuring classes.
Methods:
- Interfaces can only declare abstract methods (methods without a body). Any class implementing an interface must provide concrete implementations for all declared methods.
Fields:
- Interfaces can only have constants (public static final fields) and cannot contain instance variables.
Example:
interface Shape {
// Abstract method
double calculateArea();
}
interface Drawable {
// Abstract method
void draw();
}
class Circle implements Shape, Drawable {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
// Implementation of calculateArea from Shape interface
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
// Implementation of draw from Drawable interface
@Override
public void draw() {
System.out.println(“Drawing a circle.”);
}
}
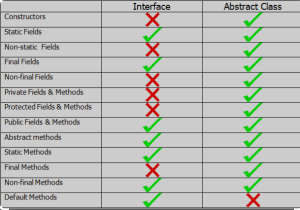
Choosing Between Abstract Classes and Interfaces:
- Use Abstract Classes:
- When you want to provide a common base class with some shared implementation.
- When you need constructors in your class hierarchy.
- When you want to declare fields (instance variables) in the base class.
- Use Interfaces:
- When you need to achieve multiple inheritance.
- When you want to define a contract for classes to implement without providing any shared implementation.
- When you want to design a lightweight, loosely-coupled system.
Key Differences
Here are some key differences between interfaces and abstract classes in Java:
Type of methods:
An interface can have only abstract methods. From Java 8, it can have default and static methods also1. An abstract class can have both abstract and non-abstract methods.
Variables:
An abstract class can have final, non-final, static, and non-static variables1. The interface has only static and final variables.
Inheritance:
An abstract class can extend another Java class and implement multiple Java interfaces1. An interface can extend another Java interface only.
Access Modifiers:
An abstract class can have public, protected, and default methods1. All methods of an interface are implicitly public.
Implementation:
Abstract classes provide a way to achieve partial abstraction (0 to 100%), whereas interfaces are used for full abstraction (100%).
Conclusion
Understanding the distinctions between abstract classes and interfaces is crucial for effective object-oriented design. Each has its strengths and use cases, and choosing the right approach depends on the specific requirements of your application.